Guide To Secure API Development
In an age powered by data and innovation, safeguarding APIs—the backbone of modern applications—is crucial. These open interfaces facilitate seamless system integration and help organizations tap into their data’s potential. Unfortunately, their very accessibility also makes them prime targets for threats.
API security vulnerabilities can lead to breaches of sensitive user data, business logic manipulation, and denial-of-service attacks, posing severe threats to organizations. Recent research indicates that only 40% of organizational APIs undergo consistent vulnerability testing, with many attacks going inadequately addressed. This underscores the critical importance of integrating API security throughout the software development lifecycle (SDLC).
This article offers a comprehensive roadmap to building APIs while ensuring that security is baked in from the start to the end of the SDLC. When you’re done, you’ll clearly understand best practices and actionable tips for holistic API security.
Summary of key secure API development concepts
The secure API development life cycle
Building secure APIs requires integrating security practices into each phase of the development process. The diagram below shows an overview of key activities across the secure API development life cycle.

#1 Requirements and planning
Secure APIs start with gathering security requirements alongside functional needs. Planning for security from the start is crucial: It’s far easier and more cost-effective to build secure APIs from the ground up than to attempt to retrofit security measures later.
The role of security in requirements gathering
The following steps ensure security while defining the functional requirements in the beginning stages of API development:
#2 Design and architecture
The design phase focuses on defining robust API architectures, platform features, and infrastructure to provide defense in depth.
Threat modeling
Threat modeling involves identifying potential threats and vulnerabilities, prioritizing risks, and defining mitigations. It is a continuous process providing insights to strengthen the planned architecture and design.
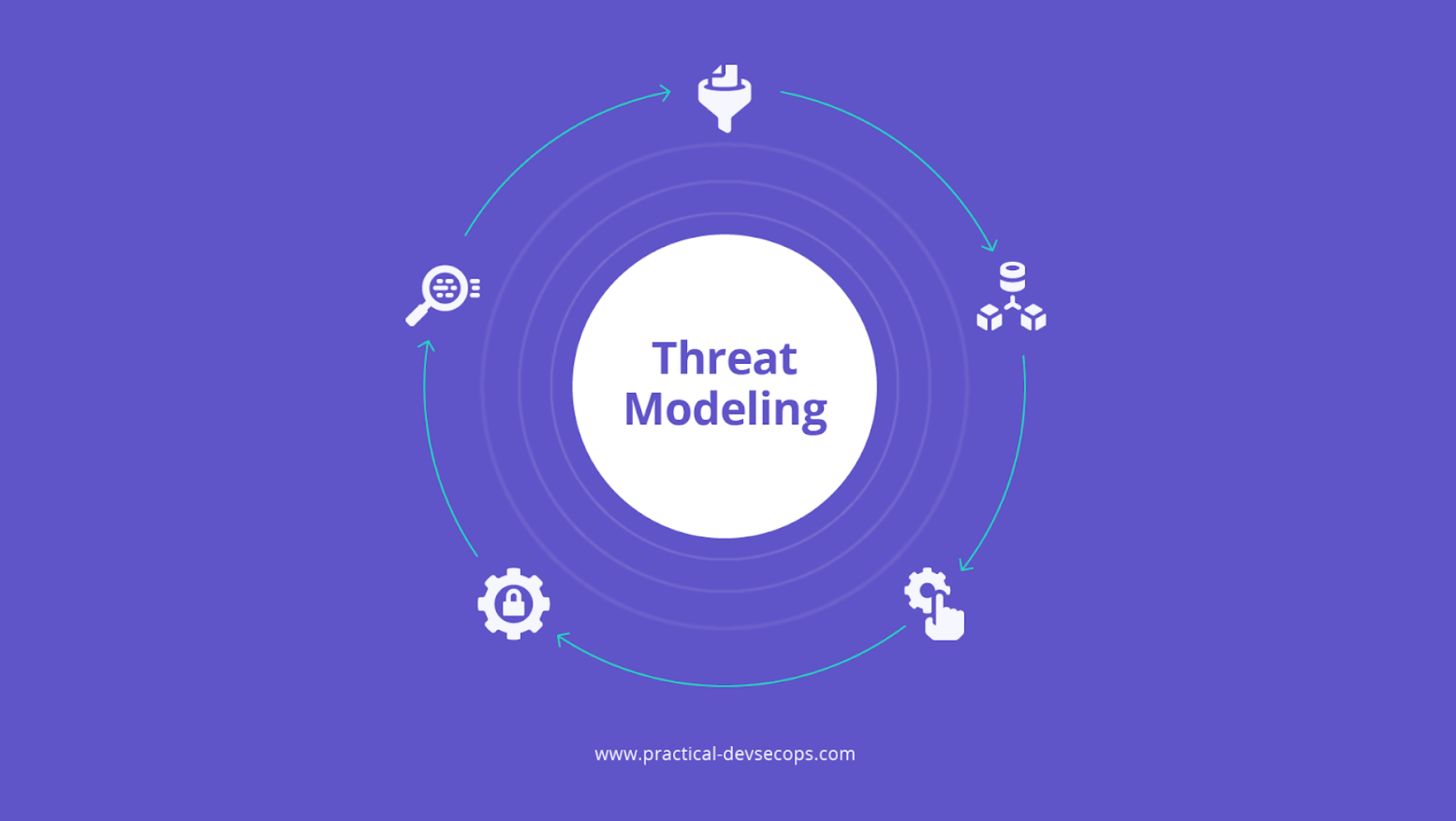
The STRIDE model—Spoofing, Tampering, Repudiation, Information Disclosure, Denial of Service, and Elevation of Privilege—is a commonly used framework for conducting threat modeling in API development.
Adopt a secure-by-design philosophy
The secure-by-design philosophy emphasizes security from the start of development rather than as an afterthought. This approach endorses principles like enabling security by default, adhering to the principle of least privilege, employing layered protections, isolating sensitive APIs, ensuring that APIs fail safely, and maintaining simplicity to avoid exploitable gaps.
Adopt a RESTful API architecture
RESTful API architecture is favored for its inherent security benefits compared to more complex SOAP-based architectures. Its fundamental constraints, such as statelessness, a client-server architecture, and a uniform interface with standardized HTTP verbs, all contribute to reducing vulnerabilities, enhancing performance, and facilitating the integration of security controls.
Tools like Impart can assist with generating documents and diagrams across the Software Development Lifecycle.
#3 Development
During development, APIs should be implemented while incorporating essential security controls directly in the code.
Secure authentication
Authentication ensures the identity of users, systems, or apps, safeguarding APIs from spoofing, tampering, and unauthorized disclosures. It’s best to use established protocols like OAuth 2.0, OpenID Connect, and JWT instead of custom methods. These standards facilitate the validation of scopes, state parameters, and specific OAuth flows while advocating cryptographic best practices such as using secure, random numbers for token generation.

Use JWT libraries instead of custom JWT creation to prevent errors. Ensure token security with encryption, proper key management (explained later on), and secret rotation. Automate the revocation of compromised or expired tokens for safer authentication.
Enforce authorization
After successful authentication, it’s crucial to manage API resource access with authorization controls. Using role-based access control (RBAC) or attribute-based access control (ABAC) ensures tailored access permissions. By following the principle of least privilege and adopting row-level security and tenant isolation in multi-tenant apps, you can greatly reduce unauthorized access risks.
{{banner-small-1="/design-banners"}}
Validate all inputs
Rigorous input validation is crucial to thwart injection attacks. Effective strategies to ensure clean, correct, and valuable data operations include adopting OWASP recommendations. Examples of these are employing allowlists over denylists, constraining data types, sanitizing inputs, and revalidating on the server side (even if client-side validation has been executed).
The following code snippet shows a basic example of validating incoming data in Python using a library like Pydantic.
The code in the try block will fail because the supplied data (age="twenty") fails the integer requirement in the Pydantic model.
Implement robust logging
Logging is indispensable for security monitoring, incident investigation, and forensics. Capture detailed authentication events, input validation failures, and API call specifics. Ensure that logs are tamper-proof, possibly through digital signatures or centralized storage, and mask sensitive fields to prevent exposure to confidential data.
Handle errors securely
Striking a balance in error handling to provide debugging context without exposing sensitive system details is vital. Employ generic error messages, classify error severity, obfuscate details, and log extensively internally for diagnostic purposes.
The following code snippet demonstrates how to craft informative yet non-leaky error messages in a Flask API.
Enable rate limiting
Rate limiting is crucial to safeguard APIs against abuse, such as brute-force login attempts and DDoS attacks. User-level, server-level, and geography-based rate limiting can be employed using various rate-limiting algorithms such as the token bucket, leaky bucket, fixed window, and sliding window algorithms to efficiently control the rate of requests.
Implement secure caching
Enhance API performance and guard against traffic surges using secure caching. Use Cache-Control: private to stop intermediaries from storing resources. Carefully handle cache keys, particularly by adding secondary keys like Vary: Cookie. This segregates cached data based on different cookie values, preventing the unintentional sharing of sensitive details among users.
Encrypt sensitive data
Employ encryption meticulously for data in transit using HTTPS and TLS and for data at rest through database and file encryption. Utilize tokenization, masking, and data minimization strategies to further bolster sensitive information security.
{{banner-small-2="/design-banners"}}
Securely store secrets
Proper secret storage is critical to prevent unauthorized access. Avoid hard-coding secrets and opt for secure storage solutions like Hashicorp Vault. Implement robust key management practices, including systematic key rotation, deployment automation, and granting least privilege access to developers for essential tasks.
Follow secure coding standards
Adhere to secure coding standards by reusing trusted libraries, utilizing parameterized queries to prevent SQL injection, removing developer comments from code before production deployment, and enforcing strict typing and input-output filtering to detect and rectify issues early in the development cycle. Many IDE and stand-alone tools can assist in a shift-left approach with security linters during development, ensuring a secure API that avoids misconfigurations like those found in the OWASP API top 10.
#4 Testing
Robust security testing is pivotal to identifying vulnerabilities before they reach production. By integrating these testing mechanisms within the CI/CD pipeline, timely feedback can be achieved, promoting a more secure development lifecycle.
Static and dynamic application security testing (SAST and DAST)
SAST and DAST form the core of automated security analysis. SAST evaluates raw source code for vulnerabilities like memory leaks without executing programs, facilitating early issue detection at code check-in time. DAST proactively assesses running applications for flaws, such as injection attacks. Incorporating tools like OWASP ZAP and Impart can bolster static and dynamic analyses, seamlessly integrating them into the CI/CD pipeline and developer IDEs for a well-rounded security assessment and timely feedback throughout development and during builds.
Runtime application self-protection (RASP)
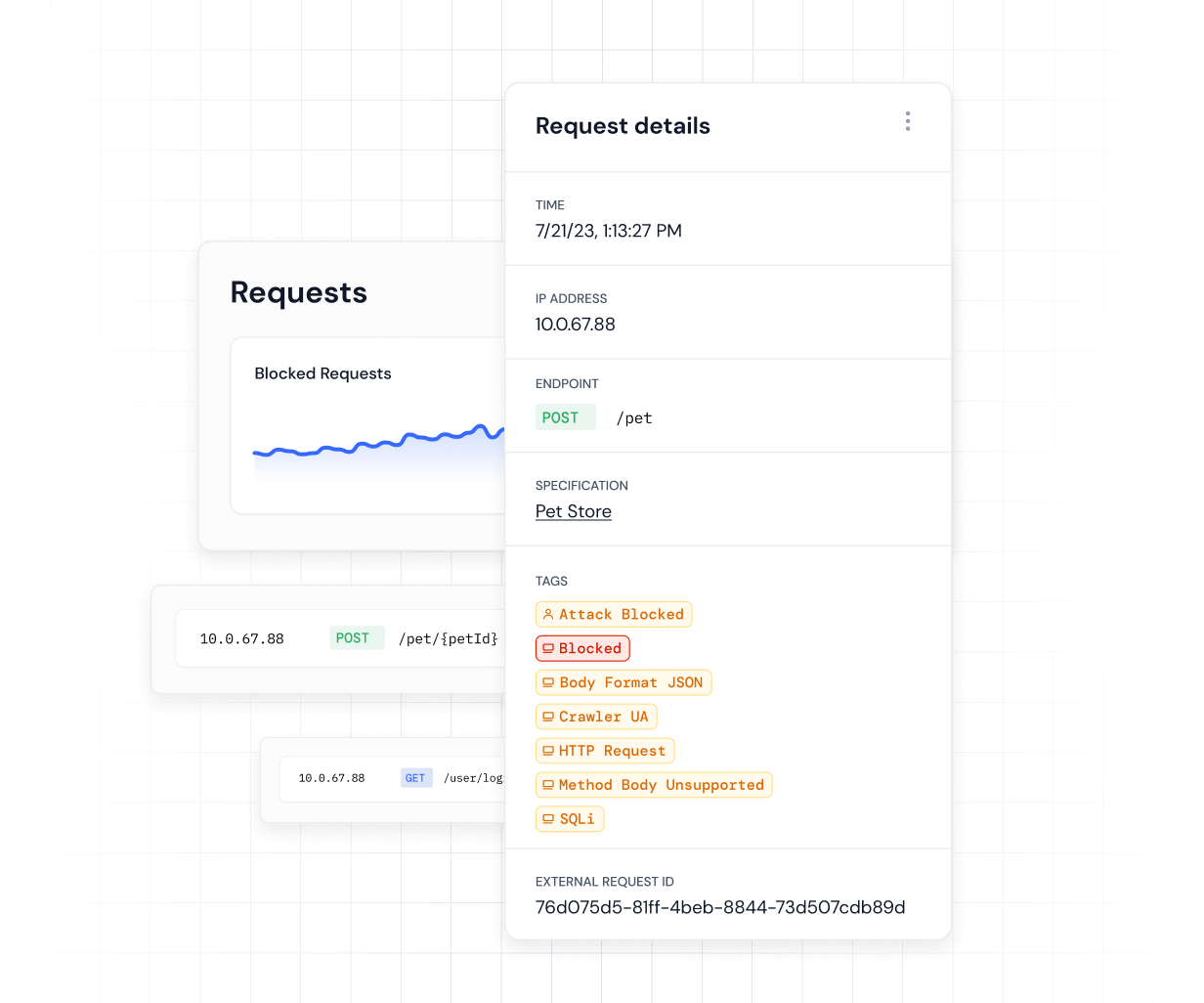
RASP focuses on safeguarding live APIs in the production environment by actively monitoring and neutralizing threats in real time, ensuring continued security post-deployment. Runtime protection can be employed using tools like Impart, which uses machine learning technology for real-time evaluations, ensuring a comprehensive framework that provides ongoing security assurance in the dynamic landscape of API threats.
Penetration testing
Utilize external penetration testers for live-fire exercises that emulate real-world attacks, mirroring likely adversaries. Scheduling periodic penetration tests on critical APIs and infrastructure throughout the year offers objective insights into exploitable gaps, aiding in promptly remedying identified vulnerabilities. This exercise is important to ensure the robustness of your API’s defenses against simulated threat attacks.
Fuzz testing
Fuzzing, a robust testing mechanism, sends unexpected or malicious data to APIs to observe behavior and uncover flaws. Attempting to crash systems or provoke unusual behaviors can flag potential vulnerabilities, contributing to mitigating unforeseen security risks that might be exploited in a real-world scenario.
#5 Deployment
The deployment phase encompasses setting up and managing infrastructure through infrastructure as code (IaC) tools such as Terraform and Ansible and securely launching APIs into production with a focus on scaling ongoing protections.
Utilizing API gateways
Utilize API gateways to route API traffic, standardizing security and enhancing performance. This enables DDoS protection via rate-limiting, reduces latency with caching, and ensures data security with authentication, authorization, and correct HTTPS/TLS configurations. Popular gateway options include Kong, Tyk, Apigee, Amazon API Gateway, and Azure API Management.
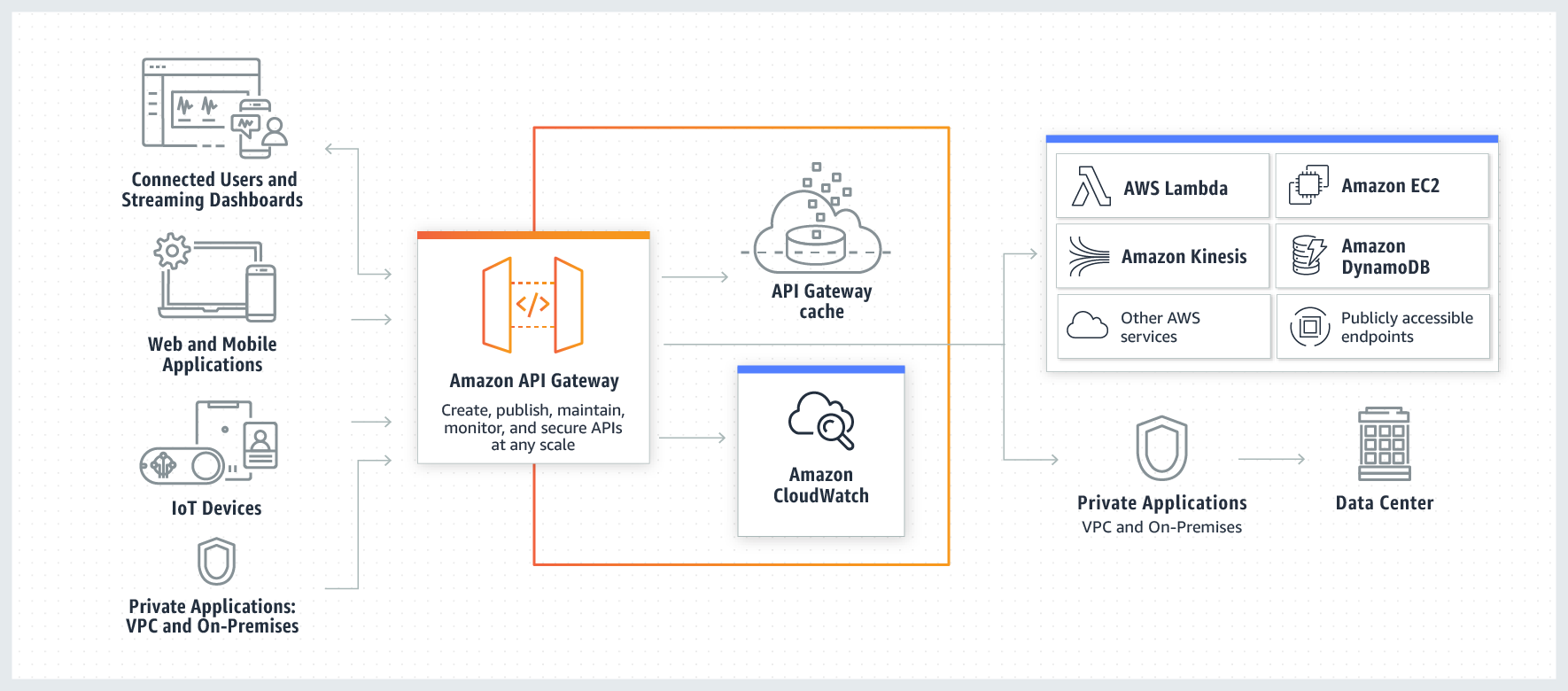
Protecting endpoints
Enhance public-facing API security by reducing exposed IPs and open ports and implementing a web application firewall (WAF) to screen traffic for threats. Block unnecessary HTTP methods, enforce up-to-date TLS and cipher suites, and maintain robust security.
Leveraging a secure CI/CD pipeline
Integrate DevSecOps in a secure CI/CD pipeline using automated tools to scan vulnerabilities in infrastructure, configurations, secrets, dependencies, and containers at every stage. Use DAST scans in build pipelines and implement IaC for consistent security baselines. Automation in these pipelines minimizes risks from manual configurations, ensuring continuous security with fewer human errors.
#6 Maintenance and monitoring
Monitoring and alerting
Real-time threat detection is enhanced by effective monitoring and logging. Monitor logins and token usage for irregularities, suggesting credential breaches or account takeovers. Track changes in roles, permissions, and groups. Monitor API traffic for odd patterns, durations, or IPs, indicating potential DDoS or brute force attempts. Analyzing metrics like response times or error rates can highlight potential denial-of-service threats. It is advisable to utilize tools like Impart to automate the monitoring and alerting of anomalies while blocking suspicious activity.
Payload inspection
Examining API payloads enhances security by revealing unknown vulnerabilities, policy breaches, and attack patterns. This involves detecting unusual API payloads, testing for injection attacks, and checking for sensitive or malicious data.
Effective payload inspection practices may encompass sampling live traffic, masking or tokenizing sensitive fields before logging, and leveraging AI techniques like anomaly detection to identify sensitive information in unstructured data flows.
API lifecycle and asset management
Optimally managing API lifecycles, including versioning and deprecation, is vital for security. Use tools to automatically identify APIs, keys, and data flows, ensuring constant inventory and preventing loss over time. Embracing API lifecycle platforms reduces security risks from outdated APIs and keys. Automated asset management further maintains a real-time view of all API assets.
Security retrospectives and continuous improvement
Regular cross-functional retrospectives on security incidents, penetration tests, and abuse scenarios are vital to suggest control enhancements. This ongoing evaluation aids in assessing the current security protocols and pinpointing areas to strengthen API security.
{{banner-large-white="/design-banners"}}
Summary
Securing APIs requires a coordinated effort encompassing people, processes, and technology. It starts with integrating security into the API design and continues throughout its lifecycle. Adopting a DevSecOps mindset is essential, and tools such as Impart simplify the process by automating key security tasks on a single cloud platform. By following the roadmap in this article, you’ll strengthen your APIs against evolving cyber threats and maximize the benefits of APIs for a secure digital transformation.
Contact Impart Security at try.imp.art for more API security tips and best practices and be sure to follow us on LinkedIn for the latest product news and updates.
Subscribe to our LinkedIn Newsletter to receive more educational content
Guide To API Security Best Practices
Learn how to protect customer data and improve security posture with 8 essential API security best practices.
API Pentesting Methodology
Learn how to scope an API, address the top five attacks, and report and retest vulnerabilities during API penetration testing.
API Attacks
Learn how API attacks, such as Broken Object Level Authorization, can lead to unauthorized access to confidential data and how to protect against them.
API Security Monitoring
Understand the best practices for monitoring your API, as well as some key features to look for when evaluating an API monitoring solution.
API Security Testing
Learn how to evaluate the security of an API and prevent common threats and vulnerabilities with twelve essential API security testing best practices.
API Security Tools
Learn how to use API security tools for offensive and defensive strategies, such as OWASP ZAP, Burp Suite, ffuf, Kiterunner, Postman, Swagger, and Im
API Security Solutions
Learn how to select a robust API security solution with features, best practices, and guidelines to ensure secure data exchange.
Secure API Development
Explore a detailed guide to API development with security at its core, covering the entire SDLC. Gain insights into best practices and practical tips for comprehensive API protection.
API Gateway Security
Learn how to secure your API gateway with 8 best practices, from authenticating users to rate limiting and hardening your apps.
OWASP Top 10 API
Learn how to prevent API security breaches with OWASP API Security Top 10 and implementing best practices for attack prevention.
API Authentication Security Best Practices
Learn how to implement robust API authentication security measures with best practices and example solutions.
API Discovery
Learn how to discover, document, and manage APIs for organization owners and developers with this article on API discovery best practices.