API Authentication Security Best Practices
Software as a service (SaaS) and microservice architectures dominate the tech landscape, and the security of application programming interfaces (APIs) is more crucial than ever. APIs form the core of many digital services, so they pose a significant security risk if not properly safeguarded.
A key defense mechanism in API security is robust authentication. This functions like airport security, rigorously checking each request to verify appropriate credentials, thereby protecting data and services from unauthorized access and potential threats.
This article delves into API authentication security best practices. We explore various authentication methods, demonstrate how to integrate these practices into systems, and discuss tools that offer comprehensive API security solutions. Rather than just listing recommendations, we provide a practical guide to implementing these strategies, empowering your organization to actively adopt these critical security measures.
Summary of API authentication security best practices
Understanding different API authentication mechanisms
API authentication serves as a critical gatekeeper, verifying identities and allowing only authorized access to your API—akin to a bank vault’s security.
Different authentication methods cater to various security needs: API keys provide basic, effective authentication for lower-risk cases, while OAuth offers heightened security by controlling access through permissions and tokens, which is ideal for secure data sharing. JSON Web Tokens (JWT) are particularly useful for single sign-on (SSO) scenarios, ensuring data integrity with digital signatures.
The choice of authentication method hinges on your specific requirements and the data sensitivity involved, ensuring that your API remains well protected.
Utilize strong authentication protocols
Implementing strong authentication protocols for APIs is critical to securing your digital assets. This section focuses on JWT, OAuth 2.0, and OpenID Connect, all of which are protocols designed for robust API security.
JWT in APIs
JWT offers a compact method to represent claims between two parties. In API authentication, JWTs enable the secure transmission of user credentials, with each token comprising a header, payload, and signature.
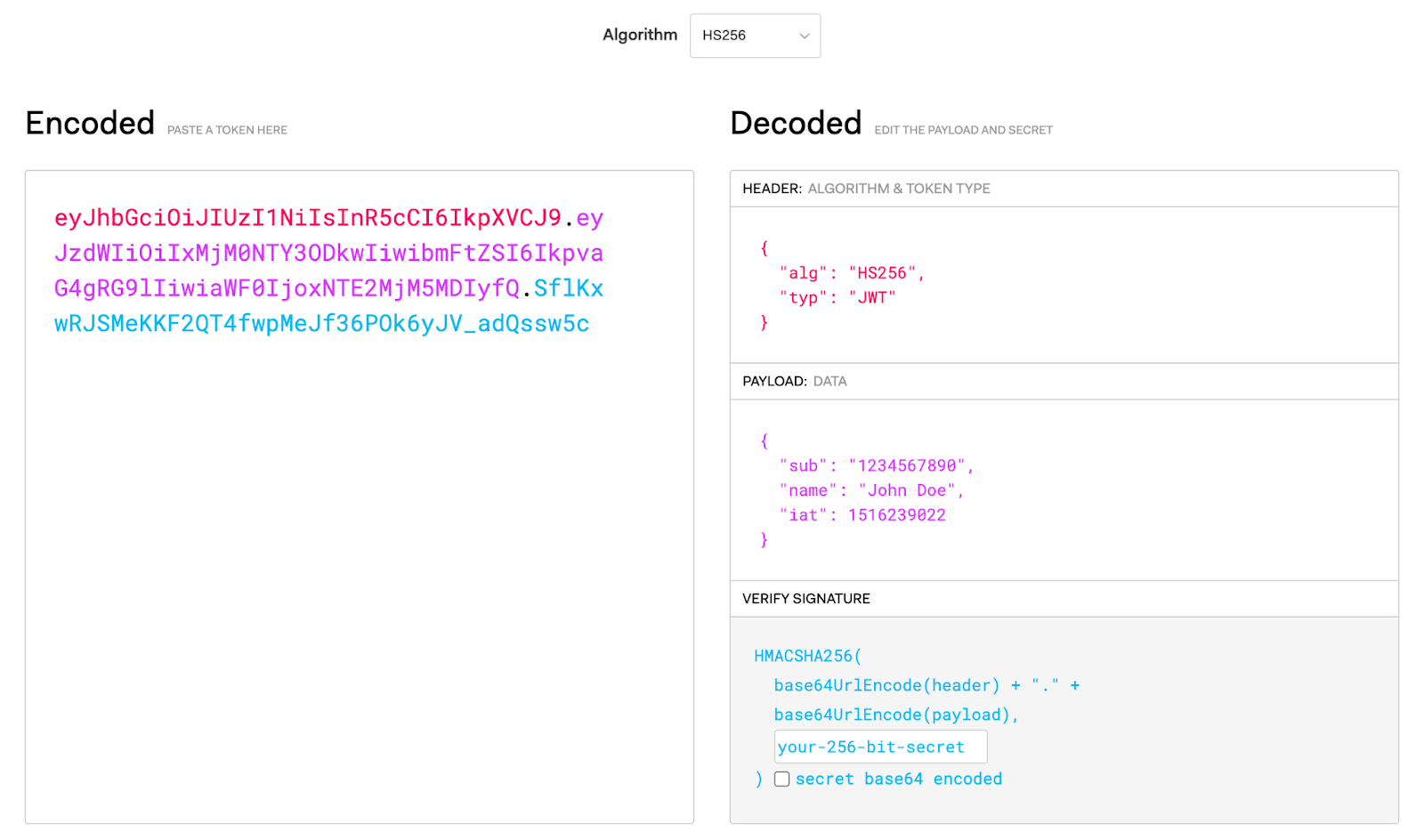
The header specifies the token type and algorithm, the payload contains user claims or information, and the signature (generated using a secret key) validates the token’s integrity. A JWT is issued and included in API requests when a user is authenticated, ensuring that only authenticated users can access the API. Here’s a Python snippet for creating a JWT:
OAuth 2.0 and OpenID Connect
OAuth 2.0 is an advanced authorization framework that allows users to authorize third-party applications to access their resources hosted on a different service. This can be achieved without exposing the user’s credentials to the third-party application. Instead, OAuth 2.0 utilizes access tokens granted by the resource owner and can be limited in scope regarding what it allows the third-party application to do.
OpenID Connect, an extension of OAuth 2.0, introduces an additional layer by adding user authentication. While OAuth 2.0 is primarily concerned with authorization (granting access to resources), OpenID Connect also verifies the user’s identity, providing information about the user’s authentication to the third-party application. This is done through an ID token, a JSON Web Token (JWT) containing authenticated user information.

Together, these protocols offer a robust solution for APIs needing secure user data access. They enable APIs to authenticate users and obtain necessary permissions to access user data while maintaining the integrity and confidentiality of user credentials. This approach is especially critical when APIs interact with other services and must ensure secure access and reliable user authentication.
Token management and secure storage
Securely handling tokens is crucial. Store tokens securely (preferably in a secure vault using a solution like Hashicorp Vault or AWS secrets manager), monitor their usage, and implement expiration. This practice minimizes the risk of token theft or misuse. Regularly rotating tokens and applying strict access controls add an extra layer of security.
Implement multi-factor authentication (MFA)
Integrating multi-factor authentication (MFA) into API authentication significantly enhances security while maintaining a quality user experience. MFA methods range from SMS codes and email to biometric verification and authenticator apps, each of which has security and convenience trade-offs. For example, authenticator apps offer more security than SMS, which can be compromised by SIM swap attacks.
To add MFA to your API, update the authentication process to introduce MFA challenges after initial credential verification. Consider this Python code example for implementation guidance:
In this implementation, once the primary credentials are confirmed, an MFA challenge is initiated, requiring users to submit an additional verification factor.
It’s important to balance security and the user experience, possibly through adaptive MFA, which varies authentication steps based on the assessment of risk (such as unusual login locations). Integrated API security services can help by offering real-time monitoring and adjusting authentication dynamically in response to detected risks.
Effective MFA integration aims to enhance security without burdening users, achieving a secure yet user-friendly authentication process.
{{banner-small-1="/design-banners"}}
Securely manage API keys
API key management is a cornerstone of API security, involving processes like generating, distributing, and rotating API keys. A well-managed API key lifecycle ensures that your application’s access remains secure, yet efficient. Let’s dive into strategies for API key generation, distribution, and rotation.
Secure generation
The foundation of API key security lies in its generation. Using cryptographically strong algorithms is essential. For instance, Python’s secrets module is a good choice for generating secure, random tokens, which can serve as API keys. Here’s a simple way to generate a secure API key in Python:
Controlled distribution
Distributing API keys securely is just as crucial. It’s a good practice to transfer them over encrypted channels and ensure that they are only accessible to authorized individuals.
Regular rotation
Periodically rotating API keys minimizes the risk of misuse. An effective strategy is to set expiration dates for keys and automate the renewal process. This can be easily managed with secrets management tools, which facilitate the secure storage of API keys and their rotation.
Integrating these strategies into your API key management process ensures a robust defense against unauthorized access, keeping your API interactions secure and trustworthy. Remember: The key to security is not just in the creation of API keys but also in their consistent and vigilant management.
Enforce encryption for API communications
Securing data in transit is a key best practice for API security, with encryption—notably, Transport Layer Security (TLS)—playing a crucial role. Think of TLS as a protective tunnel ensuring the safe passage of API calls, guarding against the interception and manipulation of sensitive authentication data like tokens and secrets.

Implementing TLS involves obtaining a valid SSL/TLS certificate from a trusted authority and confirming the server’s authenticity to clients. To ensure secure API calls, especially in Python, using libraries like requests is recommended for making API calls over secure channels.
This snippet ensures that the connection to your API is encrypted. Notice the verify=True parameter? That’s your guarantee that the TLS implementation is active and functional.
However, TLS is not a set-and-forget solution. It requires regular updates to counter evolving threats. Certain platforms can be invaluable here, offering real-time monitoring and automatic updates to ensure that your encryption standards are always up to date.
Whitelist trusted origins
Cross-origin resource sharing (CORS) is a security feature that allows you to specify which origins can access resources on your server. In the context of an API, it prevents malicious domains from making unauthorized requests.
Setting up a CORS policy involves specifying which domains can make requests (allow-listing). Here’s a simple way to implement this in Python:
In this snippet, the Flask framework is used to create a simple API. The flask_cors extension then allows us to define a CORS policy, specifying https://yourtrusteddomain.com as the trusted origin.
Maintaining an origin allowlist is an ongoing process. As your application evolves, you might need to add or remove domains. Regularly reviewing and updating this list ensures that only legitimate sources can interact with your APIs. Implementing a meticulous CORS strategy ensures that your API’s communication channels are as secure as a fortress, accessible only to those with the right credentials.
Update and patch authentication services
Maintaining robust API security necessitates regular updates and the patching of authentication services, especially when using external providers like Amazon Cognito, Azure AD B2C, or OAuth companies. These services frequently update to address vulnerabilities and enhance security, and implementing these updates is essential to protect against new threats.
Patching should be done cautiously, with testing in a controlled environment to prevent issues in live systems. This process helps avoid risks like downtime and compatibility problems. Regular updates are not merely reactive—they proactively fortify your API’s defenses by equipping authentication services with the latest security protocols, thereby reducing the likelihood of breaches.
Automation can play a key role in streamlining this process. For example, using scripts to automatically monitor and alert about new updates from these services ensures timely awareness and implementation of crucial security patches, enhancing overall API security.
{{banner-small-2="/design-banners"}}
Monitor and log access for anomaly detection
Monitoring and logging are crucial for protecting API authentication systems by detecting anomalies. Utilizing tools that integrate seamlessly into various environments enhances real-time API monitoring. Impart provides a platform that offers monitoring, firewall, testing, and remediation in one cloud platform, aiding in discovering API inventory and responding to security risks by analyzing runtime API and CI/CD developer activities.
When monitoring authentication, record both successful and failed attempts, noting timestamps, source IP addresses, and user agent strings. This data helps identify potential breaches or attacks. Set up systems to alert on unusual activities, like repeated failed logins from one IP or unexpected traffic spikes from uncommon geographic areas.
Regularly review these logs and alerts to adapt your security strategies. This proactive analysis is key to identifying and mitigating threats before they develop further.
Embracing zero-trust architecture (ZTA)
Zero trust is a strategy based on the principle “never trust, always verify.” It involves treating each API request with skepticism, regardless of its source. Key to this is understanding your API inventory thoroughly, a task made easier with Impart’s API Security Cloud, which helps identify shadow or zombie APIs.
Central to zero trust is stringent access control, employing strong authentication and authorization (such as OAuth 2.0) to ensure that only verified requests access APIs. An example is Akamai Technologies, which had a 2009 data breach that emphasized the need for application-level security. Akamai created a system for secure internal application access, reducing reliance on traditional VPNs by incorporating solutions like Soha Systems for role-based access control.
In a zero-trust framework, monitoring and logging are vital. Platforms like Impart play a key role here, enabling real-time detection and response to anomalies, which is essential for rapid breach identification and resolution.
Rate-limit and throttle requests
Rate limiting, a method to cap the number of requests a user can make within a specific timeframe, is essential for preventing API abuse, including brute-force and DDoS attacks. The challenge lies in setting appropriate limits: too strict, and you inconvenience legitimate users; too lenient, and you risk security breaches. The right limit depends on your API’s nature and typical user behavior. For example, APIs for financial transactions might need tighter limits than those for less sensitive data like weather updates.
To determine the optimal rate limit, analyze your API’s average legitimate usage and set the threshold slightly higher. This strategy safeguards regular users’ experience while deterring potential attacks. Implementing rate limits in Python can be straightforward: Libraries like Flask-Limiter allow for easy integration of rate limiting into your API routes, effectively balancing user accessibility and security.
Adaptive throttling takes rate limiting a step further, adjusting in real time based on current traffic and threat intelligence. This method is particularly effective during DDoS attacks, where request rates can spike unexpectedly.
Implementing adaptive throttling requires deeply understanding your API’s typical traffic patterns. Machine learning algorithms can analyze these patterns and adjust thresholds dynamically, ensuring that throttling kicks in when needed but doesn’t disrupt normal operations.
Certain platforms can offer rate limiting and adaptive throttling, using advanced analytics to distinguish between normal and abnormal traffic, thus optimizing API performance while securing it against threats.
Use API gateways for enhanced security
An API gateway centralizes your infrastructure’s entry point, streamlining authentication for enhanced uniform security. In microservice architectures, it ensures consistent authentication across services using standards like OAuth 2.0 or OpenID Connect.
Beyond simplifying authentication, gateways like APIGee or Amazon API Gateway, combined with security solutions like Impart, add layers of security through detailed logging and real-time monitoring of API traffic. They also enforce rate limits and access controls, bolstering your API security. Acting as both a guard and filter, the gateway allows only authenticated and authorized requests, reducing unauthorized access and bolstering API ecosystem security.
{{banner-large-white="/design-banners"}}
Conclusion
In the complex but manageable world of API authentication, adopting best practices is crucial for enhancing digital security. Effective strategies, like using OAuth, API keys, and API gateways, are key to strengthening an organization’s security posture.
Remember, however, that complacency introduces risk in API security, making continuous adaptation and learning vital. Ensuring strong authentication, strict access control, and regular API monitoring is essential for maintaining a secure API environment, and that requires ongoing effort.
Integrated API security solutions are invaluable, providing tools for API inventory management, risk detection, and real-time protection, thus moving security from a reactive to a proactive stance.
Adopting these authentication best practices and utilizing the right resources is essential for developing a robust API security framework—a critical step for organizations in the digital age. This journey, with the right tools and knowledge, is fundamental to protecting our digital infrastructure.
Contact Impart Security at try.imp.art for more API security tips and best practices and be sure to follow us on LinkedIn for the latest product news and updates.
Subscribe to our LinkedIn Newsletter to receive more educational content
Guide To API Security Best Practices
Learn how to protect customer data and improve security posture with 8 essential API security best practices.
API Pentesting Methodology
Learn how to scope an API, address the top five attacks, and report and retest vulnerabilities during API penetration testing.
API Attacks
Learn how API attacks, such as Broken Object Level Authorization, can lead to unauthorized access to confidential data and how to protect against them.
API Security Monitoring
Understand the best practices for monitoring your API, as well as some key features to look for when evaluating an API monitoring solution.
API Security Testing
Learn how to evaluate the security of an API and prevent common threats and vulnerabilities with twelve essential API security testing best practices.
API Security Tools
Learn how to use API security tools for offensive and defensive strategies, such as OWASP ZAP, Burp Suite, ffuf, Kiterunner, Postman, Swagger, and Im
API Security Solutions
Learn how to select a robust API security solution with features, best practices, and guidelines to ensure secure data exchange.
Secure API Development
Explore a detailed guide to API development with security at its core, covering the entire SDLC. Gain insights into best practices and practical tips for comprehensive API protection.
API Gateway Security
Learn how to secure your API gateway with 8 best practices, from authenticating users to rate limiting and hardening your apps.
OWASP Top 10 API
Learn how to prevent API security breaches with OWASP API Security Top 10 and implementing best practices for attack prevention.
API Authentication Security Best Practices
Learn how to implement robust API authentication security measures with best practices and example solutions.
API Discovery
Learn how to discover, document, and manage APIs for organization owners and developers with this article on API discovery best practices.