Guide To API Gateway Security Best Practices
Microservices and APIs are becoming a staple of web applications and software development. More and more applications are shifting from a monolithic architecture to more agile and dynamic microservices.
This shift turns a large piece of software into smaller pieces of components, there is a need for a central system for coordination. This is where API gateways come into play. An API gateway is responsible for coordinating, routing, load balancing, and securing the communication between an end user and the services they want to access.
As API gateways become increasingly popular, knowing how to secure those systems is essential. Even API gateways from industry leaders can have security flaws. For example, AWS’ API gateway was found to be vulnerable to issues such as HTTP request-smuggling. This leaves the API gateway open for exploitation by attackers, which would allow them to make unauthenticated requests to internal APIs and microservices, bypassing the gateway’s security.
This article will explore the top eight API gateway security best practices organizations can use to secure their resources. These best practices range from strategies and techniques to architectural styles that help protect your organization from threats.
Summary of key API gateway security best practices
The table below summarizes the eight API gateway security best practices this article will explore in more detail.
The top eight API gateway security best practices
The eight API gateway security best practices below can help organizations improve their overall security posture and mitigate the risks associated with microservices and API gateways.
#1 Securely authenticate users
Since API gateways provide the entry point to microservices, gateways should provide the authentication to those services to save time in network operations and latency.
The three most common authentication mechanisms for API gateways are the same as those used in standard API deployments:
API keys can supplement these techniques. However, according to NIST 800-204, using API keys as an authentication method should be avoided when accessing sensitive data. It is easy for API keys to get leaked, stolen by hackers, or generally mishandled, especially if the API gateway has vulnerabilities that allow an attacker to do so.
Regardless of the authentication mechanism (e.g., stateless or stateful, JWT, OAuth, etc.), teams must implement it securely. For example, in using JWTs, one of the most popular authentication methods for APIs, the token must expire as soon as possible after determining the possible duration of the session created. Also, the secret used to sign the JWTs must be stored securely and not hardcoded in source code. Instead, developers can use env files, or secrets management tools like Hashicorp or Doppler.
For reference, here is an example of code that hardcodes a JWT secret.
#2 Avoid unauthorized access
Like authentication, authorization to microservices should be provisioned through the API gateway. The gateway should be responsible for correctly deciding which authenticated user has access to the requested microservices and forwarding the requests to those microservices.
It is possible to create a network of API gateways with a hierarchical structure when necessary. An initial API gateway can forward a request for access to a microservice to an API gateway closer to that microservice (often called a micro gateway). Then, the micro gateway can decide on the permission to access the microservice. The initial API gateway provides general access, while the secondary gateway gets a more detailed "look" at the request based on the authorization method used. This prevents a single point of failure compared to using one API gateway.
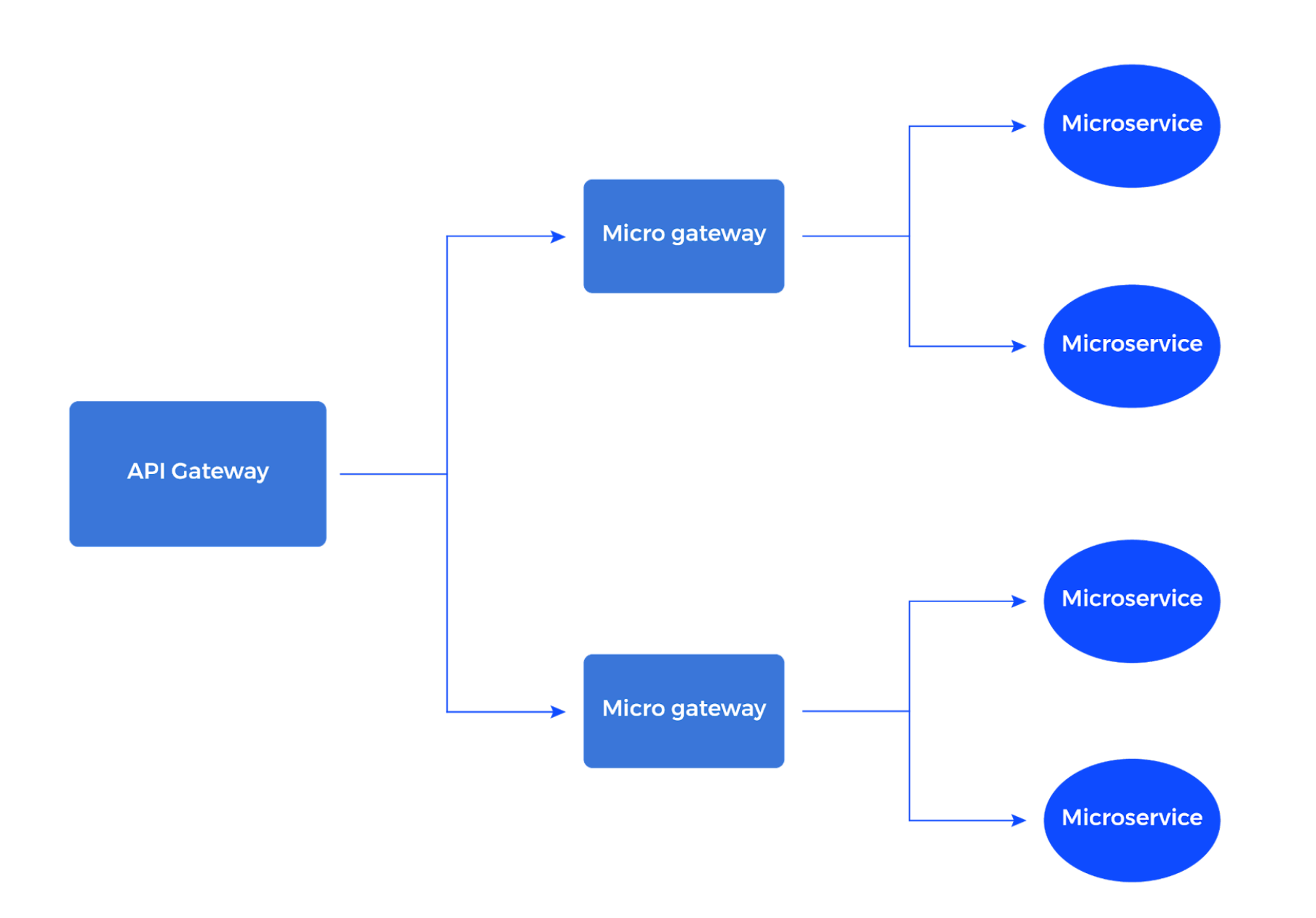
No matter what architectural style is followed regarding the authorization, it is important not to allow the users to bypass the "wall" of an API gateway.
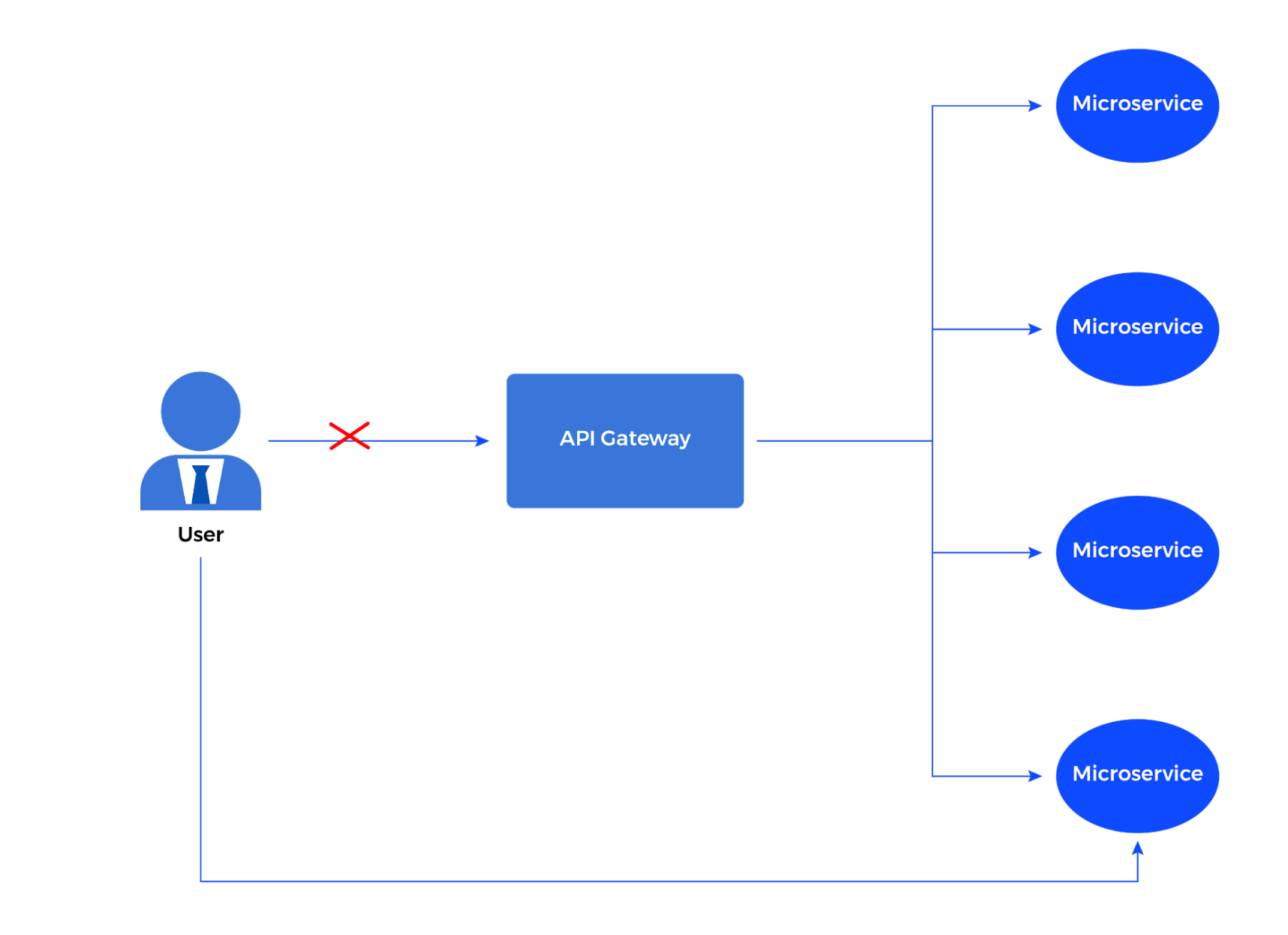
This happens when an attacker discovers a microservice exposed to the internet (or when an attacker positions themselves within the internal network) and makes direct unauthenticated calls to it before forwarding the request through the gateway, similar to how attackers bypass Cloudflare WAF protection.
API gateways should provide authorization for all microservices and be the first step of each user request. Teams can implement mutual authentication between the request source and the microservice to avoid anonymous, unauthenticated direct calls to microservices.
{{banner-small-1="/design-banners"}}
#3 Encrypt traffic
As all things connected to the Internet should be secured by strong cryptographic protocols for encrypting communication, an API gateway should not be an exception. The use of SSL/TLS is a fundamental part of web security, hence also API security.
The Client-to-API gateway and the API gateway-to-service communication should use SSL/TLS encryption to prevent Man-in-the-middle attacks on both external and internal traffic.
#4 Enforce rate limiting
To maintain the availability and stability of the gateway, throttling mechanisms must be implemented.
To implement the rate-limiting mechanisms, one should consider the requirements and specifications of the infrastructure and the application that runs on that infrastructure. The amount of API and microservice requests allowed should be based on the context of the request itself.
For example, a vast number of requests on the payment endpoint of an e-shop microservice should be handled differently than a large number of requests on its homepage. The first case could be an attempt of DoS, race condition, fuzzing, etc., while the second could be a sudden burst of activity by one or different sources.
This also requires a sense of contextual behavior and contextual categorization of the API endpoints from the defender’s point of view. AI and machine-learning models that can quickly identify malicious behaviors based on context typically enable this functionality.
Lastly, mechanisms should also be based on session characteristics such as the session key and IP address.
#5 Validate and sanitize input
“Never trust user input” is a fundamental security principle. Sanitizing user input helps enforce this principle in practice. While AI is getting better and better at recognizing patterns of malicious users, its methods are never bulletproof. Thus, there is a need for human intervention to actively make sure that no user should be trusted when handling sensitive operations by developing filters and sanitizing mechanisms in the source code.
Sanitizing sensitive characters such as double quotes, single quotes, brackets, etc., can prevent simple yet dangerous vulnerabilities such as SQL injections (SQLi), cross-site scripting (XSS), etc. An input filter should first occur in a microservice's entry point, i.e., at the API gateway level.
Also, preventing user input on decision-making input fields used by the backend system is an important step. For example, a popular API product that offered IP source data was utilizing an API gateway for load-balancing requests to the backend API. This API gateway’s rate-limit mechanism could be bypassed by changing the value of a header to a seemingly “innocent” value, enabling attackers to use the premium version of the product free of charge. Hence, it is essential to also consider the logical aspect of an operation based on a field that attackers could change and abuse.
{{banner-small-2="/design-banners"}}
#6 Log and monitor system traffic and events
Even if all other security measures have been taken, an organization needs to log and monitor the traffic of its systems. In the case of API gateways, securely monitoring both the gateway and the microservices behind it should be utilized to catch anomalous activity early on.
Real-time traffic analysis and response help prevent threats on the spot. Threats such as injection attacks, denial of service (DoS) attacks, crashes, and errors must be logged for further inspection and archive tracking. The traffic analysis and any behavioral analysis by intrusion detection systems (IDS) should be based on a normal baseline behavior that a normal user would have.
The results of all these monitoring practices should be aggregated on a centralized dashboard, where they can be observed so humans can take action when necessary.
#7 Harden your apps and infrastructure
Effective API gateway security includes hardening associated infrastructure and applications. Key infrastructure and system hardening techniques that organizations should implement include:
- Keeping software up-to-date with the latest patches
- Set up proper firewall rules
- Encrypt local storage
- Remove unnecessary libraries
- Using strong passwords for system users
These methods either try to prevent cyber attacks from reaching the gateway or minimize the damage done by attackers, once they can grasp leverage off the system.
#8 Write and publish quality API documentation
Developers, users, and business partners should have a reference point for the API gateway and microservices usage. One can achieve this by maintaining up-to-date documentation for all parties needing it.
One may think this isn’t a security best practice, as it does not directly prevent attacks. However, understanding how an API gateway operates from a developer's point of view is essential to add new functionality and integrate new features securely. Also, through complete API documentation, a security engineer can detect security issues more easily by viewing the requirements for each request.
Dynamic Open API specification generation tools help developers and organizations go through the process of documenting APIs and API gateways by generating the necessary information required for users and developers, as well as catching any security issues.
{{banner-large-white="/design-banners"}}
Conclusion
API gateways are a powerful tool organizations can use to make their API deployments more efficient. As microservices and API gateways continue to grow in popularity, it becomes increasingly important and urgent for organizations to mitigate API gateway security risks. By leveraging this article's eight API gateway security best practices, teams can reduce risk and improve their overall security posture.
Contact Impart Security at try.imp.art for more API security tips and best practices and be sure to follow us on LinkedIn for the latest product news and updates.
Subscribe to our LinkedIn Newsletter to receive more educational content
Guide To API Security Best Practices
Learn how to protect customer data and improve security posture with 8 essential API security best practices.
API Pentesting Methodology
Learn how to scope an API, address the top five attacks, and report and retest vulnerabilities during API penetration testing.
API Attacks
Learn how API attacks, such as Broken Object Level Authorization, can lead to unauthorized access to confidential data and how to protect against them.
API Security Monitoring
Understand the best practices for monitoring your API, as well as some key features to look for when evaluating an API monitoring solution.
API Security Testing
Learn how to evaluate the security of an API and prevent common threats and vulnerabilities with twelve essential API security testing best practices.
API Security Tools
Learn how to use API security tools for offensive and defensive strategies, such as OWASP ZAP, Burp Suite, ffuf, Kiterunner, Postman, Swagger, and Im
API Security Solutions
Learn how to select a robust API security solution with features, best practices, and guidelines to ensure secure data exchange.
Secure API Development
Explore a detailed guide to API development with security at its core, covering the entire SDLC. Gain insights into best practices and practical tips for comprehensive API protection.
API Gateway Security
Learn how to secure your API gateway with 8 best practices, from authenticating users to rate limiting and hardening your apps.
OWASP Top 10 API
Learn how to prevent API security breaches with OWASP API Security Top 10 and implementing best practices for attack prevention.
API Authentication Security Best Practices
Learn how to implement robust API authentication security measures with best practices and example solutions.
API Discovery
Learn how to discover, document, and manage APIs for organization owners and developers with this article on API discovery best practices.